
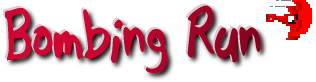
Style Guide
-
Coding style guide.
Table of Contents
Files
- C++ header files have the extension .hpp.
- C++ source files have the extension .cpp.
Indentation
- 1 tab per indent. You can change the tab settings in your editor so that the code appears indented to your liking.
- Try not to use right-aligned 'short' comments. If you must, use spaces to align them, not tabs. The rule is, "tabs to indent, spaces to align".
- 1 tab != 8 spaces. This is an assumption that is not always true.
- Do not mix tabs and spaces in your indentation!
Naming
- Follow the Java naming convention (ClassName, methodName(), variableName).
- Prefix data members of classes with the an underscore to avoid conflicts with corresponding query methods. e.g. _variableName, variableName().
Braces
-
Always use braces for the body of ifs and loops, even when they only contain one statement.
if (condition)
single_statement;
if (condition) {
single_statement;
}
-
Place your braces on the same line as your if, else, for, while and do statement, "Java style".
if (condition)
{
...
}
else
{
...
}
if (condition) {
...
} else {
...
}
-
Put a space between your if statement and your opening brace.
if (condition){
...
}
if (condition) {
...
}
Using Artistic Style
Artistic Style is an automatic C/C++/Java
code formatter. This is the configuration file required to
format your code to the above convention:
mode=k&r
brackets=attach
indent=tabs=1
brackets=break-closing-headers
Source Control Indentation
If source code style differences become a problem, it may
be desirable to implement automatic code reindentation and
formatting upon CVS check ins.